See also
A Jupyter notebook version of this tutorial can be downloaded here
.
Discriminated Single Shot Readout#
The notebook will show how to run a readout calibration experiment and fit a discriminator with a linear discriminant analysis. This experiment is sometimes called multi-state discrimination.
[1]:
from functools import partial
from typing import Literal
import matplotlib.pyplot as plt
import numpy as np
from qcodes.parameters import ManualParameter
from sklearn.metrics import ConfusionMatrixDisplay
import quantify_core.data.handling as dh
from quantify_core.analysis.readout_calibration_analysis import ReadoutCalibrationAnalysis
from quantify_scheduler import Schedule
from quantify_scheduler.enums import BinMode
from quantify_scheduler.gettables import ScheduleGettable
from quantify_scheduler.operations.gate_library import Measure, Reset, Rxy
[2]:
import json
import rich # noqa:F401
import quantify_core.data.handling as dh
from quantify_scheduler.device_under_test.quantum_device import QuantumDevice
from utils import initialize_hardware, run # noqa:F401
Setup#
In this section we configure the hardware configuration which specifies the connectivity of our system.
The experiments of this tutorial are meant to be executed with a Qblox Cluster controlling a transmon system. The experiments can also be executed using a dummy Qblox device that is created via an instance of the Cluster
class, and is initialized with a dummy configuration. When using a dummy device, the analysis will not work because the experiments will return np.nan
values.
Configuration file#
This is a template hardware configuration file for a 2-qubit system with a flux-control line which can be used to tune the qubit frequency. We will only work with qubit 0.
The hardware connectivity is as follows, by cluster slot: - QCM (Slot 2) - \(\text{O}^{1}\): Flux line for q0
. - \(\text{O}^{2}\): Flux line for q1
. - QCM-RF (Slot 6) - \(\text{O}^{1}\): Drive line for q0
using fixed 80 MHz IF. - \(\text{O}^{2}\): Drive line for q1
using fixed 80 MHz IF. - QRM-RF (Slot 8) - \(\text{O}^{1}\) and \(\text{I}^{1}\): Shared readout line for q0
/q1
using a fixed LO set at 7.5 GHz.
Note that in the hardware configuration below the mixers are uncorrected, but for high fidelity experiments this should also be done for all the modules.
[3]:
with open("configs/tuning_transmon_coupled_pair_hardware_config.json") as hw_cfg_json_file:
hardware_cfg = json.load(hw_cfg_json_file)
# Enter your own dataset directory here!
dh.set_datadir(dh.default_datadir())
Data will be saved in:
/root/quantify-data
Quantum device settings#
Here we initialize our QuantumDevice
and our qubit parameters, checkout this tutorial for further details.
In short, a QuantumDevice
contains device elements where we save our found parameters. Here we are loading a template for 2 qubits, but we will only use qubit 0.
[4]:
quantum_device = QuantumDevice.from_json_file("devices/transmon_device_2q.json")
qubit = quantum_device.get_element("q0")
quantum_device.hardware_config(hardware_cfg)
meas_ctrl, _, cluster = initialize_hardware(quantum_device, ip=None)
/usr/local/lib/python3.9/site-packages/quantify_scheduler/backends/types/qblox.py:1220: ValidationWarning: Setting `auto_lo_cal=on_lo_interm_freq_change` will overwrite settings `dc_offset_i=0.0` and `dc_offset_q=0.0`. To suppress this warning, do not set either `dc_offset_i` or `dc_offset_q` for this port-clock.
warnings.warn(
/usr/local/lib/python3.9/site-packages/quantify_scheduler/backends/types/qblox.py:1235: ValidationWarning: Setting `auto_sideband_cal=on_interm_freq_change` will overwrite settings `amp_ratio=1.0` and `phase_error=0.0`. To suppress this warning, do not set either `amp_ratio` or `phase_error` for this port-clock.
warnings.warn(
Schedule definition#
[5]:
def readout_calibration_sched(
qubit: str,
prepared_states: list[int],
repetitions: int = 1,
acq_protocol: Literal[
"SSBIntegrationComplex", "ThresholdedAcquisition"
] = "SSBIntegrationComplex",
) -> Schedule:
"""
Make a schedule for readout calibration.
Parameters
----------
qubit
The name of the qubit e.g., :code:`"q0"` to perform the experiment on.
prepared_states
A list of integers indicating which state to prepare the qubit in before measuring.
The ground state corresponds to 0 and the first-excited state to 1.
repetitions
The number of times the schedule will be repeated. Fixed to 1 for this schedule.
acq_protocol
The acquisition protocol used for the readout calibration. By default
"SSBIntegrationComplex", but "ThresholdedAcquisition" can be
used for verifying thresholded acquisition parameters.
Returns
-------
:
An experiment schedule.
Raises
------
NotImplementedError
If the prepared state is > 1.
"""
schedule = Schedule(f"Readout calibration {qubit}", repetitions=1)
for i, prep_state in enumerate(prepared_states):
schedule.add(Reset(qubit), label=f"Reset {i}")
if prep_state == 0:
pass
elif prep_state == 1:
schedule.add(Rxy(qubit=qubit, theta=180, phi=0))
else:
raise NotImplementedError(
"Preparing the qubit in the higher excited states is not supported yet."
)
schedule.add(
Measure(qubit, acq_index=i, bin_mode=BinMode.APPEND, acq_protocol=acq_protocol),
label=f"Measurement {i}",
)
return schedule
SSRO with single side band (SSB) integration#
[6]:
states = ManualParameter(name="states", unit="", label="Prepared state")
states.batch_size = 400
states.batched = True
readout_calibration_sched_kwargs = dict(
qubit=qubit.name, prepared_states=states, acq_protocol="SSBIntegrationComplex"
)
# set gettable
ssro_gettable = ScheduleGettable(
quantum_device,
schedule_function=readout_calibration_sched,
schedule_kwargs=readout_calibration_sched_kwargs,
real_imag=True,
batched=True,
)
# set measurement control
meas_ctrl.gettables(ssro_gettable)
[7]:
num_shots = 1000
state_setpoints = np.asarray([0, 1] * num_shots)
# replace the get method for the gettable in case the cluster is a dummy
if "dummy" in str(cluster._transport):
from fake_data import get_fake_ssro_data
ssro_gettable.get = partial(get_fake_ssro_data, num_shots=num_shots)
meas_ctrl.settables(states)
meas_ctrl.setpoints(state_setpoints)
ssro_ds = dh.to_gridded_dataset(meas_ctrl.run("Single shot readout experiment"))
ssro_ds
Starting batched measurement...
Iterative settable(s) [outer loop(s)]:
--- (None) ---
Batched settable(s):
states
Batch size limit: 400
[7]:
<xarray.Dataset> Size: 48kB Dimensions: (x0: 2000) Coordinates: * x0 (x0) int64 16kB 0 1 0 1 0 1 0 1 0 1 0 1 ... 0 1 0 1 0 1 0 1 0 1 0 1 Data variables: y0 (x0) float64 16kB 0.01189 0.0378 0.02313 ... 0.01414 0.02356 y1 (x0) float64 16kB 0.02268 0.03666 0.007555 ... 0.003173 0.03313 Attributes: tuid: 20241017-131148-633-d0a379 name: Single shot readout experiment grid_2d: False grid_2d_uniformly_spaced: False 1d_2_settables_uniformly_spaced: False
Fit line discriminator with linear discriminant analysis (LDA)#
[8]:
ssro_analysis = ReadoutCalibrationAnalysis(tuid=dh.get_latest_tuid())
ssro_analysis.run().display_figs_mpl()
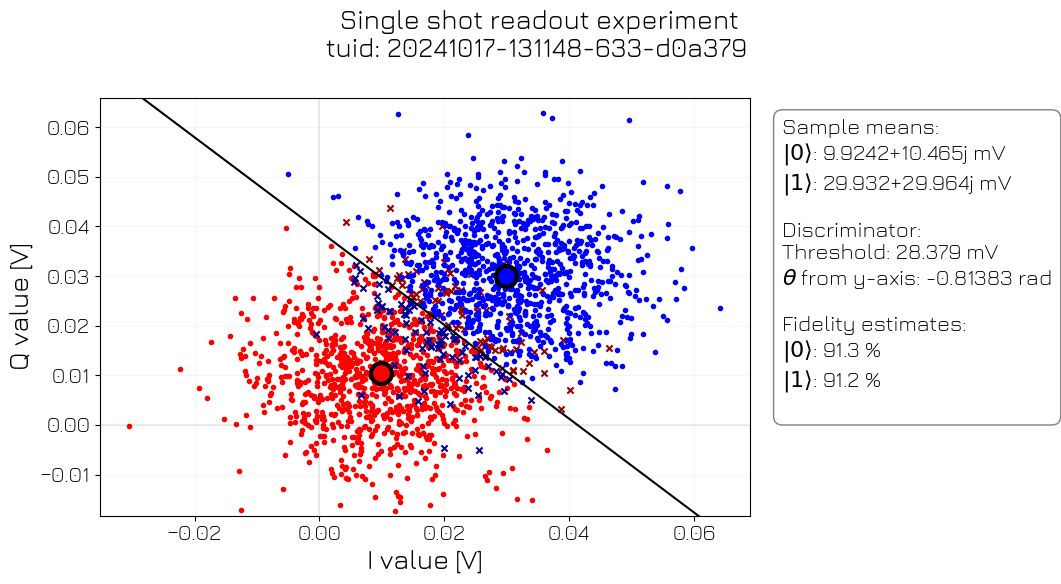
[9]:
fit_results = ssro_analysis.fit_results["linear_discriminator"].params
acq_threshold = fit_results["acq_threshold"].value
acq_rotation = (np.rad2deg(fit_results["acq_rotation_rad"].value)) % 360
qubit.measure.acq_threshold(acq_threshold)
qubit.measure.acq_rotation(acq_rotation)
SSRO with thresholded acquisition#
[10]:
disc_ssro_gettable_kwargs = dict(
qubit=qubit.name, prepared_states=states, acq_protocol="ThresholdedAcquisition"
)
# set gettable
disc_ssro_gettable = ScheduleGettable(
quantum_device,
schedule_function=readout_calibration_sched,
schedule_kwargs=disc_ssro_gettable_kwargs,
real_imag=True,
batched=True,
)
# set measurement control
meas_ctrl.gettables(disc_ssro_gettable)
[11]:
num_shots = 10_000
state_setpoints = np.asarray([0, 1] * num_shots)
# replace the get method for the gettable in case the cluster is a dummy
if "dummy" in str(cluster._transport):
from fake_data import get_fake_binary_ssro_data
disc_ssro_gettable.get = partial(get_fake_binary_ssro_data, num_shots=num_shots)
meas_ctrl.settables(states)
meas_ctrl.setpoints(state_setpoints)
disc_ssro_ds = dh.to_gridded_dataset(meas_ctrl.run("Discriminated single shot readout experiment"))
disc_ssro_ds
Starting batched measurement...
Iterative settable(s) [outer loop(s)]:
--- (None) ---
Batched settable(s):
states
Batch size limit: 400
[11]:
<xarray.Dataset> Size: 480kB Dimensions: (x0: 20000) Coordinates: * x0 (x0) int64 160kB 0 1 0 1 0 1 0 1 0 1 0 1 ... 1 0 1 0 1 0 1 0 1 0 1 Data variables: y0 (x0) float64 160kB 1.0 1.0 0.0 1.0 0.0 1.0 ... 1.0 0.0 1.0 0.0 1.0 y1 (x0) float64 160kB nan nan nan nan nan nan ... nan nan nan nan nan Attributes: tuid: 20241017-131149-991-725d30 name: Discriminated single shot readout exper... grid_2d: False grid_2d_uniformly_spaced: False 1d_2_settables_uniformly_spaced: False
[12]:
ConfusionMatrixDisplay.from_predictions(disc_ssro_ds.x0.data, disc_ssro_ds.y0.data)
plt.title("Confusion Matrix")
plt.xlabel("Measured State")
plt.ylabel("Prepared State")
[12]:
Text(0, 0.5, 'Prepared State')
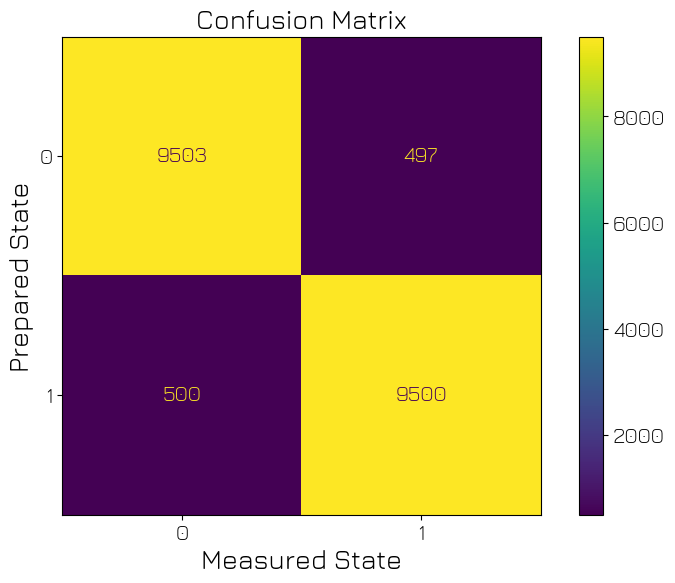
[13]:
rich.print(quantum_device.hardware_config())
{ 'config_type': 'quantify_scheduler.backends.qblox_backend.QbloxHardwareCompilationConfig', 'hardware_description': { 'cluster0': { 'instrument_type': 'Cluster', 'modules': { '6': {'instrument_type': 'QCM_RF'}, '2': {'instrument_type': 'QCM'}, '8': {'instrument_type': 'QRM_RF'} }, 'sequence_to_file': False, 'ref': 'internal' } }, 'hardware_options': { 'output_att': {'q0:mw-q0.01': 10, 'q1:mw-q1.01': 10, 'q0:res-q0.ro': 60, 'q1:res-q1.ro': 60}, 'mixer_corrections': { 'q0:mw-q0.01': { 'auto_lo_cal': 'on_lo_interm_freq_change', 'auto_sideband_cal': 'on_interm_freq_change', 'dc_offset_i': None, 'dc_offset_q': None, 'amp_ratio': 1.0, 'phase_error': 0.0 }, 'q1:mw-q1.01': { 'auto_lo_cal': 'on_lo_interm_freq_change', 'auto_sideband_cal': 'on_interm_freq_change', 'dc_offset_i': None, 'dc_offset_q': None, 'amp_ratio': 1.0, 'phase_error': 0.0 }, 'q0:res-q0.ro': { 'auto_lo_cal': 'on_lo_interm_freq_change', 'auto_sideband_cal': 'on_interm_freq_change', 'dc_offset_i': None, 'dc_offset_q': None, 'amp_ratio': 1.0, 'phase_error': 0.0 }, 'q1:res-q1.ro': { 'auto_lo_cal': 'on_lo_interm_freq_change', 'auto_sideband_cal': 'on_interm_freq_change', 'dc_offset_i': None, 'dc_offset_q': None, 'amp_ratio': 1.0, 'phase_error': 0.0 } }, 'modulation_frequencies': { 'q0:mw-q0.01': {'interm_freq': 80000000.0}, 'q1:mw-q1.01': {'interm_freq': 80000000.0}, 'q0:res-q0.ro': {'lo_freq': 7500000000.0}, 'q1:res-q1.ro': {'lo_freq': 7500000000.0} } }, 'connectivity': { 'graph': [ ['cluster0.module6.complex_output_0', 'q0:mw'], ['cluster0.module6.complex_output_1', 'q1:mw'], ['cluster0.module2.real_output_0', 'q0:fl'], ['cluster0.module2.real_output_1', 'q1:fl'], ['cluster0.module8.complex_output_0', 'q0:res'], ['cluster0.module8.complex_output_0', 'q1:res'] ] } }
[14]:
quantum_device.to_json_file("devices/")
[14]:
'devices/device_2q_2024-10-17_13-11-50_UTC.json'